#스프링 #공부 #다시시작
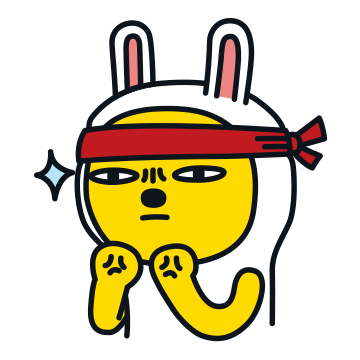
프로젝트 생성
IDE는 IntelliJ, JPA를 사용한 웹 애플리케이션 개발이 목적이다.
다음 사이트에 들어가서
+ dependencies에 lombok도 추가해주자!
Spring Boot 프로젝트가 잘 설치되었는 지 확인하기 위한 Test
package jpabook.jpashop;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class JpashopApplication {
public static void main(String[] args) {
Hello hello = new Hello();
hello.setData("hello");
String data = hello.getData(); // hello.getData()만 입력하고 Ctrl + Alt + V 누르면 자동으로 변수에 입력
SpringApplication.run(JpashopApplication.class, args);
}
}
자동 생성된 JpashopApplication을 다음과 같이 수정해준다.
package jpabook.jpashop;
import lombok.Getter;
import lombok.Setter;
@Getter @Setter // Getter Setter 어노테이션으로 클래스 변수에 쉽게 적용
public class Hello {
private String data;
}
간단하게 구현한 Hello 클래스
라이브러리 살펴보기
- 스프링 MVC
- 스프링 ORM
- JPA, 하이버네이트
- 스프링 데이터 JPA
- H2 데이터베이스 클라이언트
- 커넥션 풀
- WEB(thymeleaf)
- 로깅 & LogBack
- 테스트
View 환경 설정
1. Thymeleaf를 이용한 View 환경
package jpabook.jpashop;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello") // Get 방식으로 URL에 반환 값 전달
public String hello(Model model) { // model 이라는 이름의 모듈을 생성
model.addAttribute("data", "hello!!");
// attribute 이름은 "data"이고, 이 data의 값은 "hello!"
return "hello"; // 화면 이름
} // 이 함수가 실행되면 templates 디렉터리에 있는 hello.html 파일이 실행된다.
}
hello.html에 URL이 GET방식으로 매핑된 것을 확인할 수 있다.
<!-- resources/templates/hello.html-->
<html xmlns:th = "http://www.thymeleaf.org">
<!-- xmlns의 namespaec를 thymelea로 -->
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<p th:text = "'안녕하세요. ' + ${data}">안녕하세요, 손님</p>
<!-- p 태그는 하나의 문단을 만드는 태그 -->
<!-- data 변수는 hello 메서드에서 넘겨받은 Attribute이다 -->
</body>
</html>
2. 렌더링 없이 html 동작(정적 콘텐츠)
: main>static 디렉터리에 html 파일 생성
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello</title>
</head>
<body>
Hello
<a href="/hello"> hello </a>
</body>
</html>
H2 데이터베이스 설치
https://www.h2database.com/html/main.html
H2 Database Engine
H2 Database Engine Welcome to H2, the Java SQL database. The main features of H2 are: Very fast, open source, JDBC API Embedded and server modes; in-memory databases Browser based Console application Small footprint: around 2 MB jar file size Suppor
www.h2database.com
여기서 h2 database 엔진을 깔고 console을 실행시켜
이후에 다시 접속 시
jdbc:h2:tcp://localhost/~/jpashop
다음 코드를 JDBC URL에 입력하여 데이터베이스에 접근한다.
JPA와 DB 설정, 동작 확인
package jpabook.jpashop;
import org.springframework.stereotype.Repository;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
@Repository // 자동으로 스프링 빈에 등록. Component의 attribute이다(DB 접근하기 위한 객체)
public class MemberRepository {
@PersistenceContext
private EntityManager em;
// EntityManager에 member 객체를 저장
public Long save(Member member) {
em.persist(member);
return member.getId();
// Id만 반환하는 이유 : Command와 Query를 분리(나중에 ID를 이용해 조회를 하기 위하여)
}
// 조회하는 메서드
public Member find(Long id) {
return em.find(Member.class, id);
}
}
다음과 같이 MemberRepository 클래스를 만들어주었다.
EntityManager 객체를 만들고, save, find 함수를 만들어 객체의 입출력을 관리하였다.
테스트해보자!
package jpabook.jpashop;
import org.assertj.core.api.Assertions;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.transaction.annotation.Transactional;
import static org.junit.Assert.*;
@SpringBootTest
@RunWith(SpringRunner.class)
public class MemberRepositoryTest {
@Autowired MemberRepository memberRepository;
@Test
@Transactional
public void testMember() throws Exception{
//given
Member member = new Member();
member.setUsername("memberA");
//when
Long saveId = memberRepository.save(member); // Ctrl + Alt + V 단축키 사용
Member findMember = memberRepository.find(saveId);
//then
Assertions.assertThat(findMember.getId()).isEqualTo(member.getId());
Assertions.assertThat(findMember.getUsername()).isEqualTo(member.getUsername());
}
}
Test 코드는 MemberRepository 파일에서 Ctrl + Shift + T 를 눌러 자동 생성한다.
Junit4 로 설정 하였고, 이에 대한 오류는 dependencies에서 오류 메세지에 해당하는 부분을 주석처리하여 해결하였다.
@Transactional 어노테이션 : save 함수 처리 과정에서 에러가 나타났을 때 자동으로 Rollback 해준다.
테스트 클래스에서 난 에러인것 같고, 데이터 베이스 연결의 문제인지 의문이 든다 (ㅠㅠ)
+ (해결)
데이터 베이스 연결의 문제였고, 로컬에서 H2 데이터베이스를 키지 않고 실행해서 그렇다!
H2 데이터베이스 실행해서 Run해도 에러가 뜨길래 .. 눈물을 흘리면서 구글링
혹시나 해서 DB를 연결한 application.yml 파일을 보았는데 세미콜론과 함께 MVCC=TRUE 요 부분이 거슬렸다
검색해보니 H2가 개편되어 이 부분은 안 적는 것이 맞다고 한다.
없애니 해결
Test가 끝나면 코드를 Rollback 한다.
@Rollback(false) 어노테이션을 추가해서 Rollback을 동작하지 않을 수 있다.
jar을 빌드해서 동작을 확인하자.
> gradlew clean build
> cd build
> cd libs
> dir
> java -jar jpashop-0.0.1-SNAPSHOT.jar
다음 코드를 입력하면 8080 포트가 열리고 jpashop 파일을 확인할 수 있다.
'Framework > Spring' 카테고리의 다른 글
스프링 부트 JPA 활용 1 - 도메인 분석 설계 / 엔티티 클래스 개발 (0) | 2021.06.02 |
---|---|
[스프링 입문] 스프링 빈과 의존 관계 (0) | 2021.05.25 |
스프링 관련 의문점 다루기 (0) | 2021.02.06 |
스프링 복습 및 기초 다지기 (0) | 2021.02.05 |
인프런 스프링 입문 강의 #JPA (0) | 2021.02.02 |